Bounding boxes for object detection and localization in images
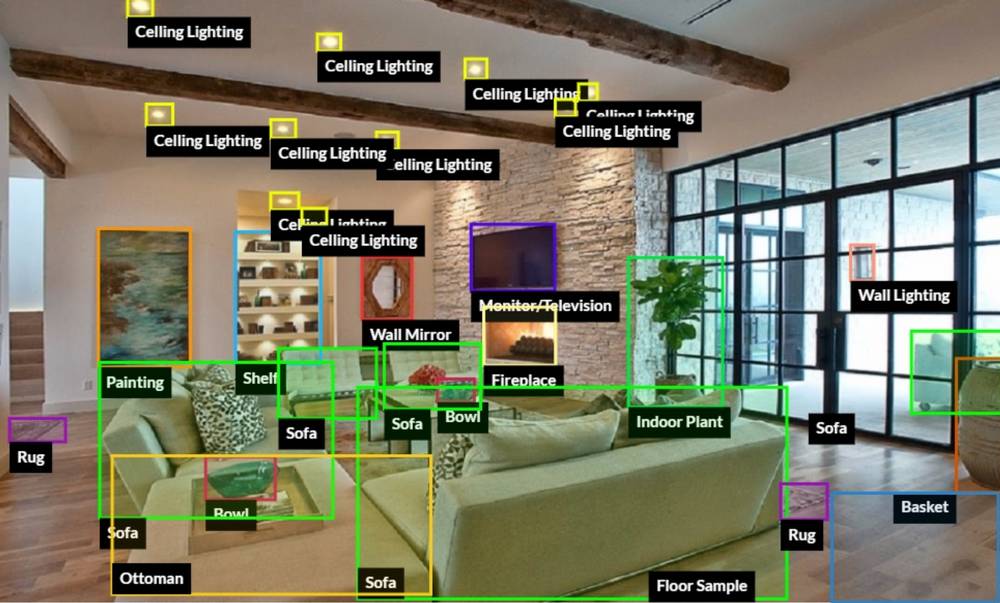
-
Create the image job as mentioned in Creating image annotation-task(Job) check the sidebar for a sample input
POST
https://api.playment.io/v0/projects/<project_id>/jobs
Parameters
project_id
: To be passed in the URL
x-api-key
: Secret key to be passed as a header
{
"reference_id": "001",
"data": {
"image_url":"https://dummyimage.com/600x400/000/fff.jpg&text=Dummy+Image+1"
},
"tag": "Sample-task",
"batch_id": "72c888f6-b365-4f27-ad57-d7841da2de0c",
"priority_weight": 5
}
{
"data": {
"job_id": "3f3e8675-ca69-46d7-aa34-96f90fcbb732",
"reference_id": "001",
"tag": "Sample-task"
},
"success": true
}
import requests
import json
"""
Details for creating JOBS,
project_id ->> ID of project in which job needed to be created
x_api_key ->> secret api key to create JOBS
tag ->> You can ask this from playment side
batch_id ->> The batch in which JOB needed to be created
"""
project_id = ''
x_api_key = ''
tag = ''
batch_id = ''
#method that can be used to call the job creation api
def Upload_jobs( DATA):
base_url = f"https://api.playment.io/v0/projects/{project_id}/jobs"
response = requests.post(base_url, headers={'x-api-key': x_api_key}, json=DATA)
print(response.json())
if response.status_code >= 500:
raise Exception("Something wrong at Playment's end")
if 400 <= response.status_code < 500:
raise Exception("Something wrong!!")
return response.json()
#method that can be used for batch creation
def create_batch(batch_name,batch_description):
headers = {'x-api-key':x_api_key}
url = 'https://api.playment.io/v1/project/{}/batch'.format(project_id)
data = {"project_id":project_id,"label":batch_name,"name":batch_name,"description":batch_description}
response = requests.post(url=url,headers=headers,json=data)
print(response.json())
if response.status_code >= 500:
raise Exception("Something wrong at Playment's end")
if 400 <= response.status_code < 500:
raise Exception("Something wrong!!")
return response.json()['data']['batch_id']
#list of frames in a single job
image_url = [ "https://example.com/image_url_1"]
#reference_id should be unique for each job
reference_id="job1"
job_data = {
'reference_id':reference_id,
'tag':api_tag,
'data':{'image_url':image_url},
'batch_id' : new_batch_id
}
#helper method to print json data structure
def to_dict(obj):
return json.loads(
json.dumps(obj, default=lambda o: getattr(o, '__dict__', str(o)))
)
print(json.dumps(to_dict(job_data)))
response = Upload_jobs(DATA=job_data)
print(response.json())
-
Fetching result as mentioned in Fetching Job Result
GET
https://api.playment.in/v0/projects/<project_id>/jobs/<job_id>
Parameters
project_id
: To be passed in the URL
x-api-key
: Secret key to be passed as a header
{
"data": {
"project_id": "",
"reference_id": "001",
"job_id": "fde54589-ebty-48lp-677a-03a0428ca836",
"batch_id": "b99d241a-bb80-ghyi-po90-c37d4fead593",
"status": "completed",
"tag": "sample_project",
"priority_weight": 5,
"result": "https://playment-data-uploads.s3.ap-south-1.amazonaws.com/sample-result.json"
},
"success": true
}
{
"data": {
"annotations": {
"cuboids": [],
"landmarks": [],
"lines": [],
"polygons": [],
"rectangles": [
{
"_id": "0e6d895e-2484-439a-b62b-d8a0afb3d190",
"attributes": {
"Overexposed image area": {
"state": "editable",
"value": "No"
},
"Rideable without rider": {
"state": "editable",
"value": "No"
}
},
"color": "rgb(0, 93, 255)",
"coordinates": [
{"x": 0.0039825622583978815, "y": 0.005589354107361727 },
{"x": 0.054049889267715416, "y": 0.005589354107361727 },
{"x": 0.054049889267715416, "y": 0.0909649321368556 },
{"x": 0.0039825622583978815, "y": 0.0909649321368556 }
],
"label": "Relevant",
"state": "editable"
},
{
"_id": "60f1c8a5-a277-4887-8b14-75c90fe31c17",
"attributes": {},
"color": "rgb(255, 0, 0)",
"coordinates": [
{ "x": 0.441582541088339,"y": 0.5907712271116603},
{ "x": 0.7829988214764708,"y": 0.5907712271116603},
{ "x": 0.7829988214764708,"y": 0.6373313968759106},
{ "x": 0.441582541088339,"y": 0.6373313968759106}
],
"label": "Object",
"state": "editable"
}
]
},
"image_url": "https://playment-data-uploads.s3.ap-south-1.amazonaws.com/clients/d3ad5a3e-b701-4f6b-a1d4-4ec2ae668ddb/projects/13c7d7dc-c79b-45e7-bbfa-1d84bfb41681/batch_upload_data/e595c2f0-141b-4f0b-8642-a9caf5e7698d/Pre-selection/relevant%20frames/20190923-123036_City__00025_F_STEREO_L_2_masked.jpg"
}
}
Result JSON Structure
Response Key | Description |
---|---|
data | object having annotations object and image_url |
data.annotations | Object having all the types of annotations namely cuboids, landmarks, lines, polygons and rectangles |
data.annotations.rectangles | List of all the bounding-box annotated in this job |
data.annotations.rectangles.[i] | one of the Object from rectangles list, having _id, label, coordinates and other attributes |
data.annotations.rectangles.[i]._id | String id of theannotated bounding box |
data.annotations.rectangles.[i].label | String class label of the annotated bounding box |
data.annotations.rectangles.[i].coordinates | List of 4 corners of the bounding box each having x,y coordinates |
data.annotations.rectangles.[i].attributes | Object having all the additional attributes associated with the annotated bounding box |
data.image_url | String URL of the image over which bounding boxes were drawn |